The following image shows the Layers palette of Illustrator. The next couple of examples will refer to the situation that this image describes:
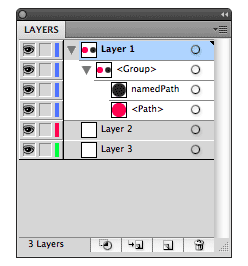
The document contains three layers. The first layer contains a group with two paths in it.
Layers in the Document
The global variable document refers to the currently active document.
Each document contains a list of layers. You can access the layers in this list through the document.layers array both by index and name.
For example to access the first layer in the document.layers array:
var firstLayer = document.layers[0]; print(firstLayer.name); // 'Layer 1'
And if we wanted to access the third layer by name:
var namedLayer = document.layers['Layer 3'];
Active Layer
All items created by Scriptographer are automatically placed in the active layer. The active layer in the screenshot above is the one that is highlighted in blue.
To access the active layer we use document.activeLayer.
var layer = document.activeLayer; print(layer.name); // Layer 1
Items in Layers
In programming we refer to items that are contained within other items as being their children. You can query any item in an lllustrator document to get its children.
To find the items in a layer, we can access its layer.children property.
For example, to move the first item in the active layer to { x: 100, y: 100 }:
var children = document.activeLayer.children; var firstChild = children[0]; firstChild.position = new Point(100, 100);
Items in Groups
We can also access the items contained in a group.
In the following example we move the second item contained in the group (the first item in the active layer) 20 points to the right:
var children = document.activeLayer.children; var group = children[0]; // the second item in the group: var child = group.children[1]; child.position += new Point(20, 0);
All items in the children array are also accessible by name. To access the child path of group called namedPath, we could replace the fifth line in the above example to:
var child = group.children['namedPath'];
Changing the Order of Items in the Layer List
Scriptographer allows you to change the order of items in the layer list in a couple of different ways. You can append an item to become a child of a group or a layer. You can also move items above or under other items as they appear in the layer list.
Appending Items to Groups and Layers
To append an item to become a child of another group or layer, we use item.appendTop(item) and item.appendBottom(item) on the group or layer and pass the item to it that we want to append. item.appendTop(item) appends the item as the first child as seen in the layer list, item.appendBottom(item) appends the item as the last child.
For example, if we would want to move the first item in the active layer to the top of third layer ('Layer 3'), we could do the following:
var firstChild = document.activeLayer.children[0]; var thirdLayer = document.layers[2]; thirdLayer.appendTop(firstChild);
The append functions work exactly the same with groups. Lets make a new path and move it into the group that is in the active layer:
var circlePath = new Path.Circle(new Point(50, 50), 25); var group = document.activeLayer.children[0]; group.appendTop(circlePath);
Moving an Item Above or Below Other Items in the Layer List
To move an item above or below another item in the layer list, we use item.moveAbove(item) and item.moveBelow(item). item.moveAbove(item) moves the item above the item that you passed to it and item.moveBelow(item) does the opposite.
For example, if we would want to move the second item of the group in the active layer above the first item, we could do the following:
var group = document.activeLayer.children[0]; var children = group.children; var firstChild = children[0]; var secondChild = children[1]; secondChild.moveAbove(firstChild);
Creating Layers
To create a new layer in the Illustrator interface we select 'New Layer' in the contextual menu of the layer palette:
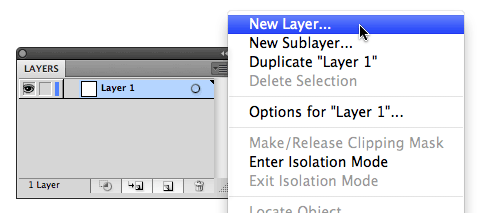
To create a new layer using Scriptographer, we call new Layer():
var layer = new Layer(); layer.name = 'Brand New Layer';
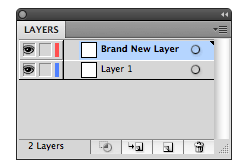
Creating Groups
To group items in a document using the Illustrator interface, you select them and select 'Group' from the 'Object' menu. Working with groups in Scriptographer is slightly different. First we make a new empty group and then we append the items to that group:
In the following example we first make an empty group, then we make two paths and append them to the group:
var group = new Group(); var firstPath = new Path.Circle(new Point(50, 50), 25); var secondPath = new Path.Circle(new Point(50, 50), 25); group.appendTop(firstPath); group.appendTop(secondPath);
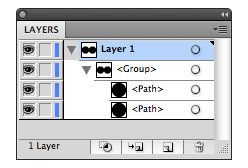