Hitting Items with the Mouse
Scriptographer allows you to easily find items that were hit by the mouse. The event object that is passed to the mouse handler functions includes the Item that was located at that position: toolEvent.item. If the item is part of a Group or a CompoundPath, then this parent is returned instead.
For example, the following mouse tool removes any item in the Illustrator document that the user clicks on:
function onMouseDown(event) { // Check whether an item was clicked on: if(event.item) { // If so, remove it: event.item.remove(); } }
Hit Tests
Using the document.hitTest(point, tolerance) function, you can find an item, curve or segment point at a specific Point in the document. The optional tolerance parameter allows you to specify the tolerance for the Hit Test in pixels.
item.hitTest(point, tolerance) works in exactly the same way, but only performs the Hit Test on the specified item.
Hit Results
When the hitTest function hits something, it returns a HitResult object, which describes the different things that were hit:
- hitResult.item: the Item that was hit.
- hitResult.segment: the Segment that was hit, if any.
- hitResult.curve: the Curve that was hit, if any.
- hitResult.parameter: the parameter on the curve that was hit, as used by various bezier curve calculations.
Let's say, for example, you wanted to make a tool that creates a circle shaped path around a segment point when a user clicks on it:
function onMouseDown(event) { var hitResult = document.hitTest(event.point); // If there is a hitResult and the hitResult // contains a segment: if(hitResult && hitResult.segment) { // Create a circle shaped path at the position // of the segment: new Path.Circle(hitResult.segment.point, 5); } }
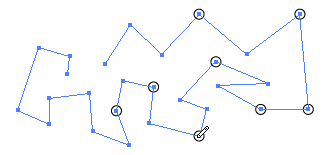
Request Options
Using the document.hitTest(point, request, tolerance) and path.hitTest(point, request, tolerance) functions, you can also specify what kind of object you're looking to hit. For example, if you only want to hit the end points of a path, you would use:
var point = new Point(50, 50); var hitResult = document.hitTest(point, 'end-points');
The different options for the request parameter are as followed:
- 'all': Hits items, anchors, handles and guides
- 'anchors': Only hits anchors i.e. segment points
- 'end-points': Only hits the first and last segment points in a path
- 'guides': Only hits guides
- 'paths': Only hits paths
- 'texts': Only hits text items
- 'selection': Only hits selected items or selected anchors
- 'all-except-guides': The same as 'all' but ignores guides
- 'all-except-guides-and-fills': The same as 'all' but ignores guides and filled areas
- 'all-except-fills': The same as 'all' but ignores filled areas
- 'all-except-handles': The same as 'all' but ignores handles
- 'all-except-guides-and-locked': The same as 'all' but ignores guides and locked items