Scriptographer offers many convenient methods for the creation of User Interfaces. A simple way for quickly outputting values while working on scripts is described in the tutorial Working with the Console. In order to interact with and report back to the user, it is recommended to create real interfaces using dialogs and palettes.
Alert Dialog
To display a message to the user, the Dialog.alert(message) function offers a simple way to display an alert dialog. It receives the string to display as the argument.
Dialog.alert('I always thought something was fundamentally wrong with the universe.');
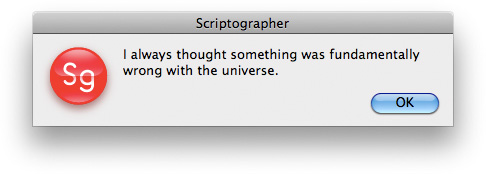
The variant Dialog.alert(title, message) additionally allows the definition of a title for the dialog. The following example also illustrates how quotes within strings need to be escaped with a preceding backslash (\) in order to not accidentally mark the end of the string.
Dialog.alert('Don't Panic', 'As long as you know where your towel is.');
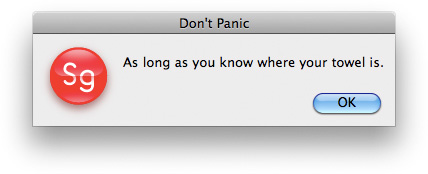
Confirm Dialog
Using the Dialog.confirm(message) function, we can ask the user a simple question that he can either confirm or cancel. If confirmed, the function returns true, otherwise false. The result can then be used to make further decisions.
var decision = Dialog.confirm('Do you want to know the Answer to the Ultimate Question of Life, the Universe, and Everything?'); if (decision) { Dialog.alert('The Answer is 42, what was the question again?'); }
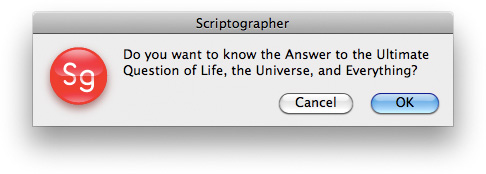
If this dialog is confirmed, the second dialog is displayed, unveiling the answer:
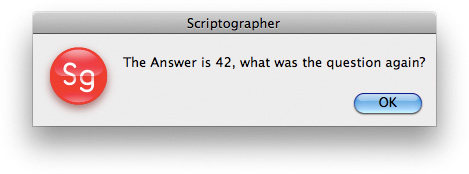
Prompt Dialog
The confirm dialog allows to ask the user simple yes/no kind of questions. For anything more complex than that, Scriptographer provides the freely configurable prompt dialog. Prompt dialogs are displayed through the Dialog.prompt(title, components) function. Just like with Dialog.alert(title, message), title defines the title of the dialog window to be displayed. The flexibility lies in components, an object containing descriptions of multiple rows of different kind of dialog components, to be displayed in the dialog. The tutorial Interface Components describes the different types of components available to create both prompt dialogs and palette windows.
Prompt dialogs and palette windows are functional twins, supporting the same components definitions. The difference lies in how the dialogs / windows are displayed: While prompt dialogs block the user interface until the user cancels or confirms, palette windows float on top of the document windows and offer options to be changed by the user at any stage of the work.
So let's look at simple example:
// First we define the dialog components var components = { firstName: { type: 'string', label: 'First Name' }, lastName: { type: 'string', label: 'Last Name' }, email: { type: 'string', label: 'Email Address' } }; // Now we bring up the dialog var values = Dialog.prompt('Please Enter Your Contact Details', components); if (values) { // Results were returned, so let's say hello to the user: Dialog.alert('Hello ' + values.firstName + ' ' + values.lastName + ', your email address is: ' + values.email); }
This brings up the following dialog, with empty text fields that can be filled in by the user:
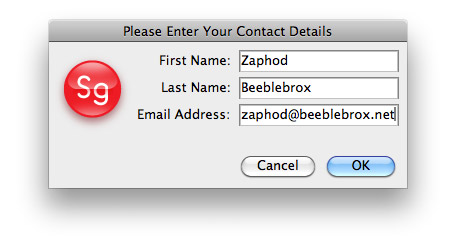
When confirmed, the dialog returns the entered values as an object that we keep in the values variable:
var values = Dialog.prompt('Please Enter Your Contact Details', components);
The results then can be looked up in the values variable under the same names as used to define the dialog components. But if the dialog was canceled, nothing is returned. So we always need to check wether the values actually contains values, before doing anything with them:
if (values) { // values contains the results, so let's say hello to the user: Dialog.alert('Hello ' + values.firstName + ' ' + values.lastName + ', your email address is: ' + values.email); }
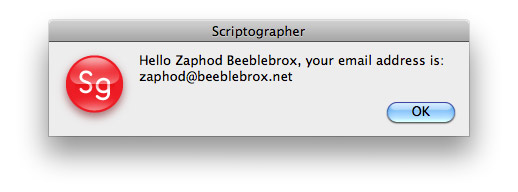
For more advanced examples and a list of all possible component types, refer to the Interface Components tutorial. In any of its examples, the creation of the palette windows can simply be replaced with a call to Dialog.prompt(title, components) instead of new Palette(title, components, values).